Multi-Domain Testing in Playwright: One Browser vs. Two Browsers
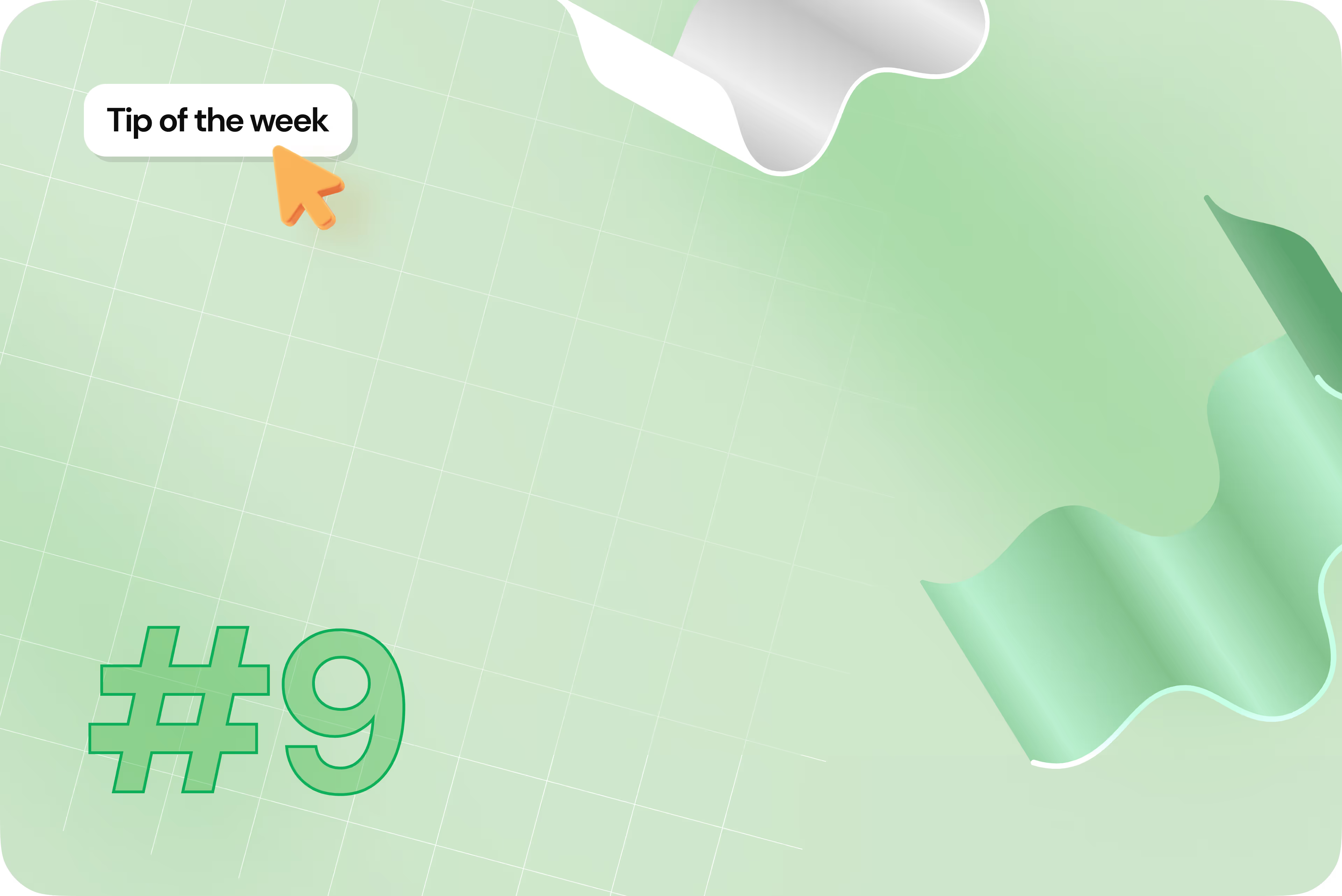
Multi-domain testing is essential when working with applications that involve authentication, cross-domain interactions, or different user roles. Playwright offers two main approaches to handle multi-domain testing:
- Using a single browser with separate contexts
- Launching two independent browser instances
Each approach has its pros and cons. This post will walk through both strategies with JavaScript examples.
Solution 1: One Browser, Separate Contexts
A single browser instance can create multiple isolated contexts, allowing separate sessions for different domains. This approach is lightweight and efficient.
Example: One Browser, Separate Contexts
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const context1 = await browser.newContext(); // First isolated context
const page1 = await context1.newPage();
await page1.goto('https://example.com');
const context2 = await browser.newContext(); // Second isolated context
const page2 = await context2.newPage();
await page2.goto('https://another-domain.com');
console.log(await page1.title(), await page2.title());
await browser.close();
})();
Solution 2: Two Browsers
For maximum isolation, you can launch two independent browser instances. This ensures completely separate environments for each domain or user role.
Example: Two Browsers
const { chromium } = require('playwright');
(async () => {
const browser1 = await chromium.launch();
const page1 = await browser1.newPage();
await page1.goto('https://example.com');
const browser2 = await chromium.launch();
const page2 = await browser2.newPage();
await page2.goto('https://another-domain.com');
console.log(await page1.title(), await page2.title());
await browser1.close();
await browser2.close();
})();
Comparison Table

Summary
Both methods have their advantages. If you need to test different sessions in a lightweight way, using a single browser with multiple contexts is the best option. However, if you require complete isolation, using two browser instances is the way to go.
Choose the approach that best fits your testing needs!