Clicking at an Offset Inside an Element
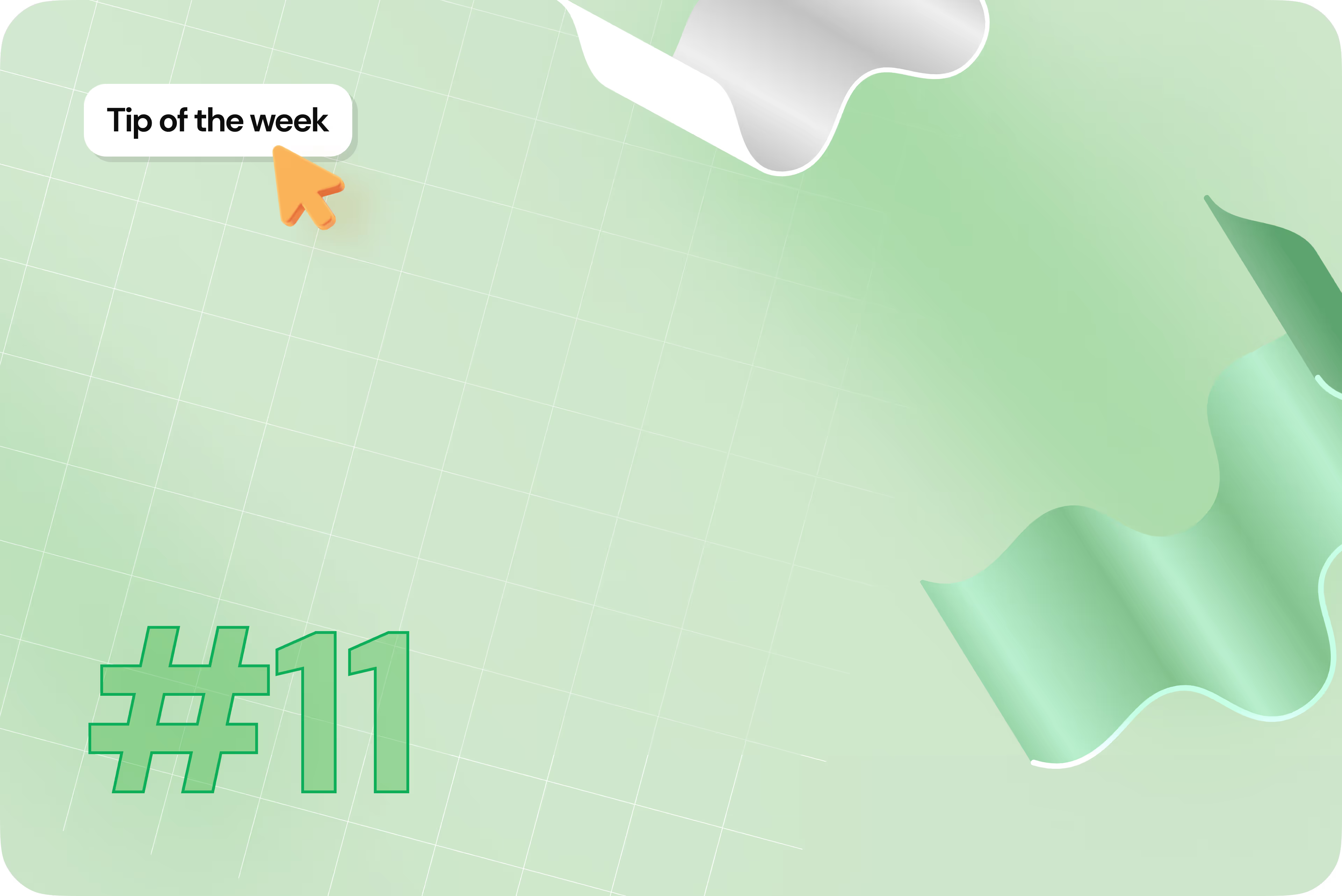
Intro
Sometimes, clicking on the center of an element just isn’t enough. You may need to click at a specific offset within the element—like clicking the top-left corner of a canvas or a particular icon inside a larger container. Playwright makes this possible using the position option in the click() method. But there’s a catch.
Solution
The position option allows you to define the X and Y coordinates within the bounding box of the target element:
await page.locator('selector').click({ position: { x: 10, y: 5 } });
This tells Playwright to click 10 pixels right and 5 pixels down from the top-left corner of the element. However, the click must remain inside the element’s bounding box, or Playwright will throw an error.
If you want to click outside the element or calculate a precise location relative to some logic (e.g., based on screen layout or element dimensions), you’ll need to use the Mouse API instead.
Example
Here’s how you can use the Mouse API to click with a custom offset from the element’s center—even if it goes beyond the bounds of the element:
const element = await page.locator('selector');
const box = await element.boundingBox();
if (box) {
const centerX = box.x + box.width / 2;
const centerY = box.y + box.height / 2;
// Add offset to center
const offsetX = 30;
const offsetY = -10;
await page.mouse.click(centerX + offsetX, centerY + offsetY);
} else {
throw new Error('Element bounding box not found');
}
This gives you full control over the click coordinates on the page—perfect for testing custom UI elements, game UIs, or visual tools.
Summary
When you need to click inside an element at a specific position, use the position option with click(). But if you need more control—or want to click outside the element’s bounds—fall back to the Mouse API. It gives you pixel-perfect control and can help you avoid limitations tied to the element’s bounding box.
Happy testing! 🧪